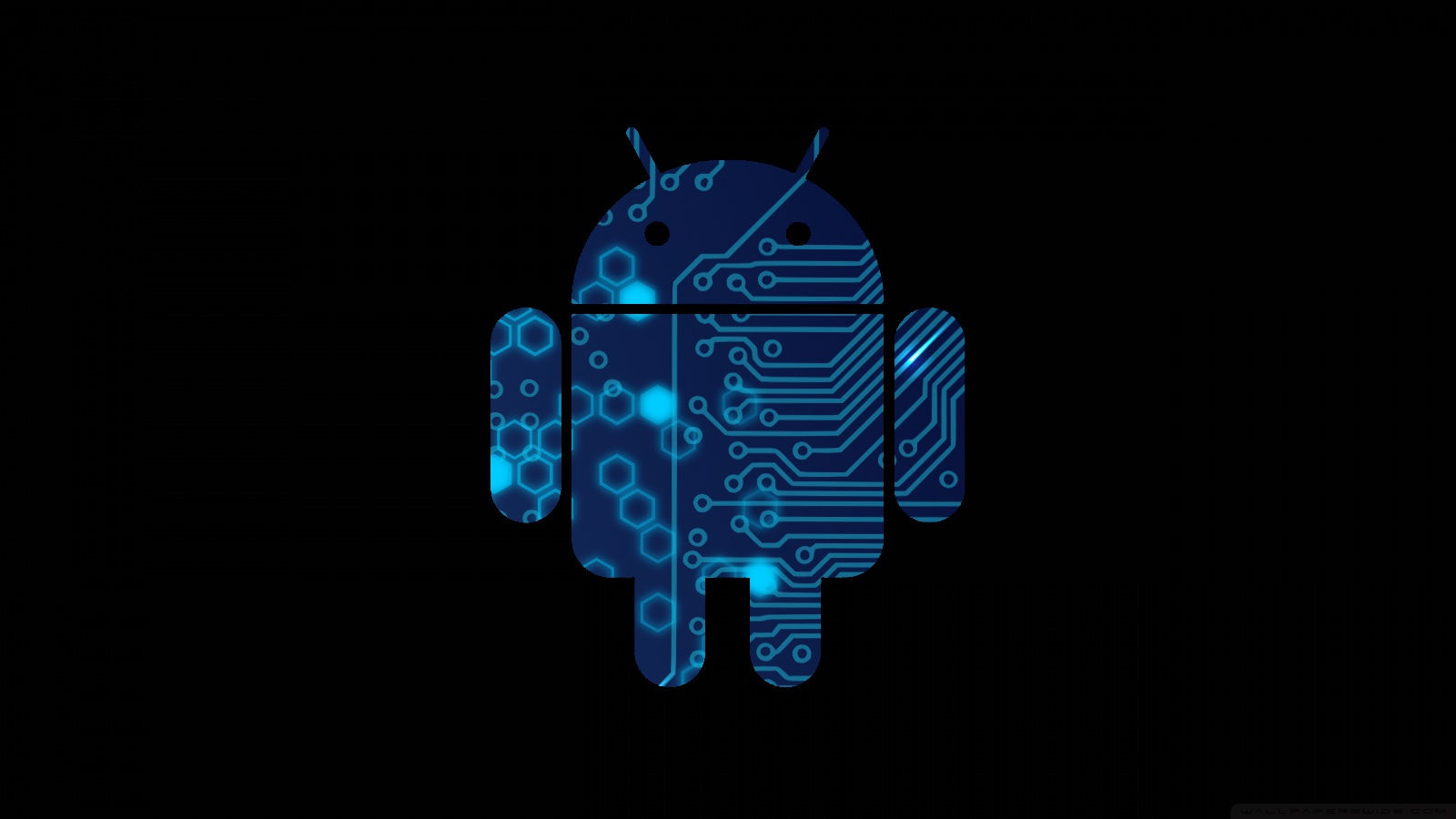
Arduino and ESP-32 Gearbox
On the Arduino deep dive, Ms. Isabel Mendiola, and Mr. Peter Haydock taught us the different features of Arduino Uno and ESP-32. In the first week, we worked with TinkeCAD circuits to simulate the different sensors and components using Arduino Uno as the controller. I already know how to work with Arduino, but since day 1 I started to learn new things like connecting an LCD display or use a gas sensor.
In the second week (which is my favorite), we started to work with wireless communication like Wi-Fi and Bluetooth. For these protocols, we worked with ESP-32. I have experience working with that microcontroller, but I never worked with HTML web pages or with voice recognition for Bluetooth connection.
Arduino Uno
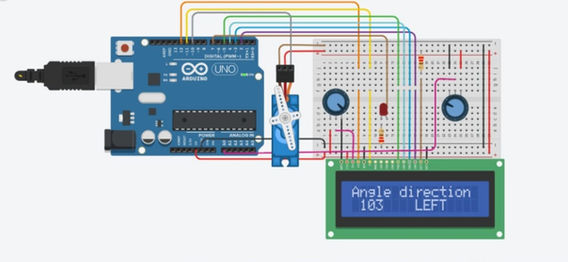
Servo control code
#include <LiquidCrystal.h>
#include <Servo.h>
int potpin1=A0;
int servalue;
LiquidCrystal lcd(12,11,5,4,3,2);
Servo myservo;
void setup(){
Serial.begin(9600);
lcd.begin(16,2);
myservo.attach(10);
pinMode(7, OUTPUT);
}
void loop (){
servalue=analogRead(potpin1);
servalue=map(servalue,0,1023,0,180);
myservo.write(servalue);
Serial.println("Servo1");
Serial.println(servalue);
lcd.setCursor(0,0);
lcd.print("Angle direction");
delay (300);
//lcd.scrollDisplayRight();
//lcd.clear();
lcd.setCursor(1,1);//0 is character, 1 is second row
lcd.print(servalue);
delay (300);
if(servalue<=90){
digitalWrite(7,LOW);
lcd.setCursor(8,1);
lcd.print("LEFT ");
}
if(servalue>=90){
digitalWrite(7,HIGH);
Serial.println("LED");
lcd.setCursor(8,1);
lcd.print("RIGHT");
}
//lcd.clear();
}
ESP-32 Intelligent house
Based on the simulation excercises, we used the ESP-32 to create a webserver to display data from our sensors. It was designed a code in which the microcontroller established a connection with our private network. I had issues trying to run the code updates because I lost my Wi-Fi and I had to use my mobile data. I controlled a fan using a temperature sensor. I designed an HTML website but I couldn't add a background or better styles.
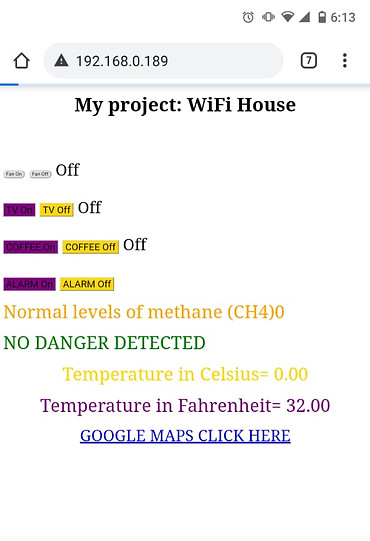
Intelligent house code
#include <WiFi.h>
#include <GAS_MQ4.h>
const char* ssid = "D5DABC";
const char* password = "L21503750317528";
int MQ4SensorValue = 0;
int valor=0;
int fan=5;
int buzzer=2;
int ledred=0;
int ledwhite=4;
#define sensor 32
const int sensort=36;
float tempc;
float tempf;
float volts; volts=analogRead(sensor);
volts=(volts*90.0)/4095; //4095
tempc=volts;
tempf=(volts*1.8)+32;
Serial.print(tempc);
Serial.println(" C ");
Serial.print(tempf);
Serial.println(" F ");
delay(1000);
if(tempc>15.0){
digitalWrite(fan,HIGH);
}
else{
digitalWrite(fan,LOW);
}
MQ4SensorValue = analogRead(32);
delay(1000);
Serial.print("MQ4 Sensor Analog Reading ");
Serial.println(MQ4SensorValue);
if (MQ4SensorValue > 800) {
Serial.println("Warning CH4 or other gas detected.");
//tone(buzzer,1000);
digitalWrite(buzzer,HIGH);
//digitalWrite(fan,HIGH);
}
else {
//noTone(buzzer);
Serial.println("NO WARNING");
digitalWrite(buzzer,LOW);
//digitalWrite(fan,LOW);
Serial.println(" ");
// Check if a client has connected
WiFiClient client = server.available();
if (!client) {
return;
} // Read the first line of the request
String request = client.readStringUntil('\r');
Serial.println(request);
//client.flush();
// Match the request
client.println("<meta http-equiv=\"refresh\" content=\"3\" >");if(MQ4SensorValue > 800){
client.println("<body><p style=\"font-size:27px;color:green\"> High levels methane (CH4)"+ String(MQ4SensorValue)+"</p>");
client.println("<body><p style=\"font-size:27px;color:green\"> THE ALARM IS ACTIVATED");
}
else if (MQ4SensorValue < 800){
client.println("<body><p style=\"font-size:27px;color:orange\"> Normal levels of methane (CH4)"+ String(MQ4SensorValue)+"</p>");
client.println("<body><p style=\"font-size:27px;color:green\"> NO DANGER DETECTED");
}
client.println("<body><p style=\"font-size:27px;Text-align: center;color:gold;\"> Temperature in Celsius= "+String(tempc)+"</p>");
client.println("<body><p style=\"font-size:27px;Text-align: center;color:purple;\"> Temperature in Fahrenheit= "+String(tempf)+"</p>");
client.print("<html><body>");
client.print("<a href=\http://www.googlemaps.com/\><center>GOOGLE MAPS CLICK HERE""</center></a>"); //ADD WEBSITE
client.print(" ");
client.println("</html>");
delay(1);
Serial.println("Client disconnected");
Serial.println("");
}
}
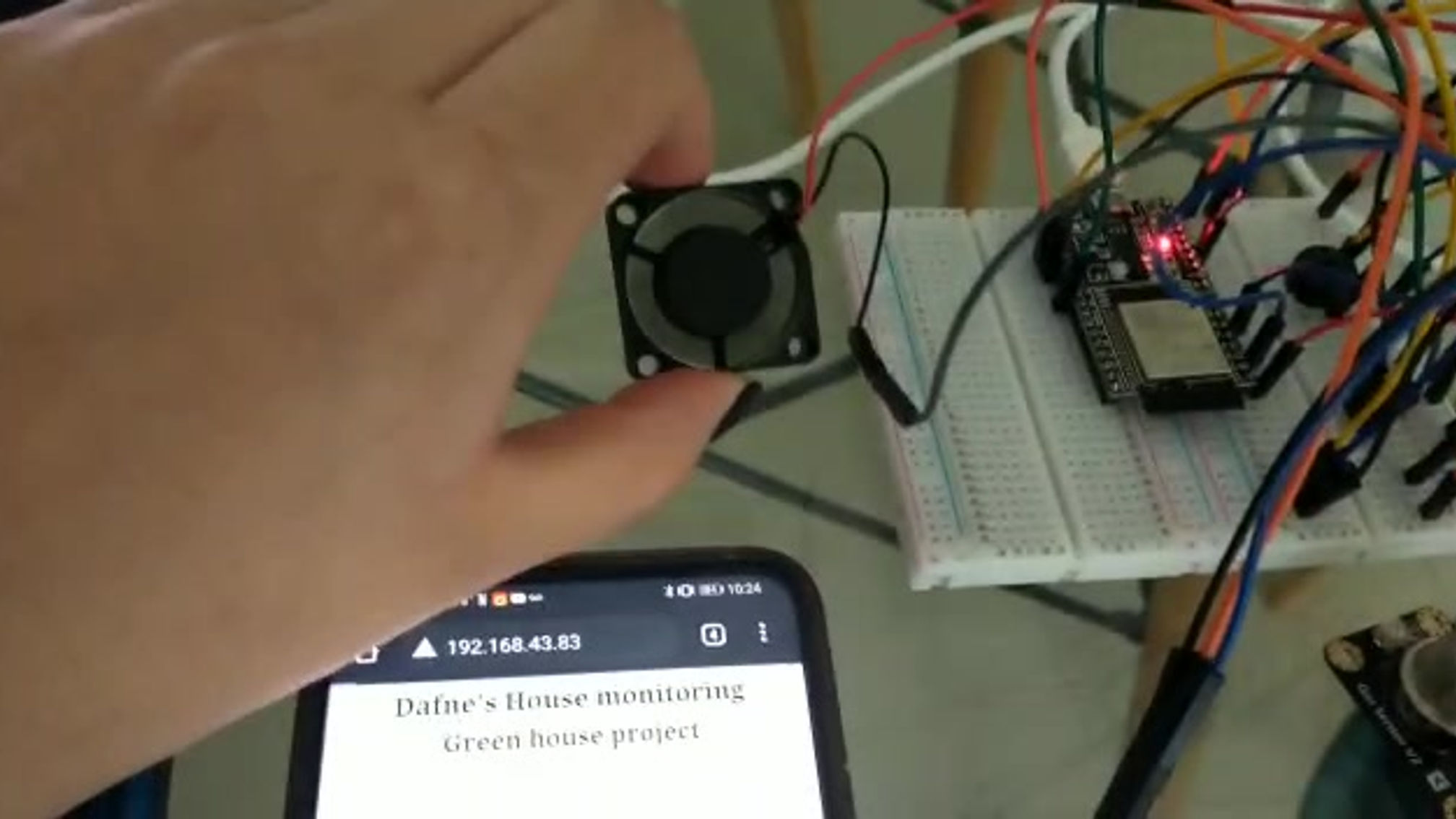
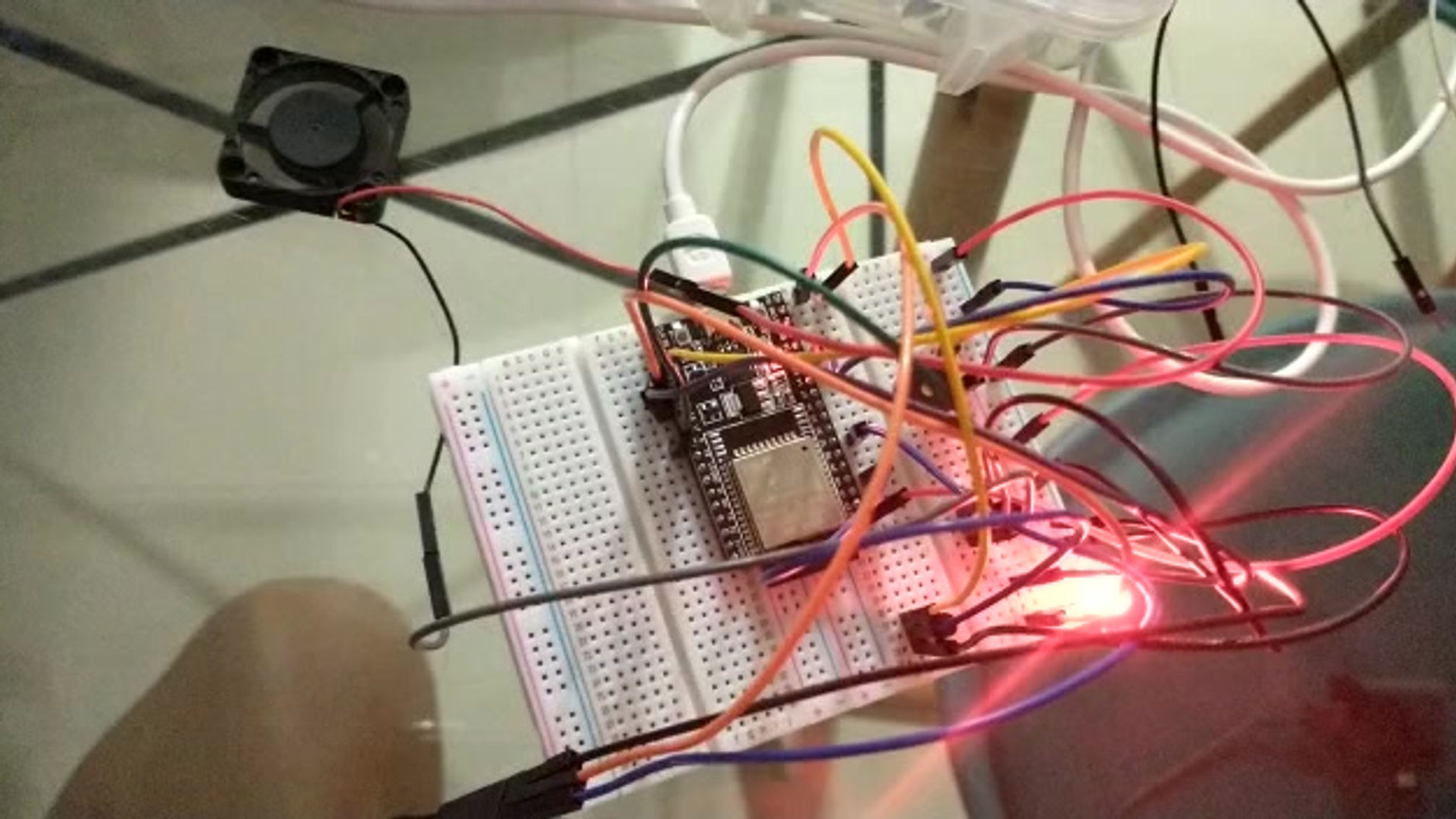
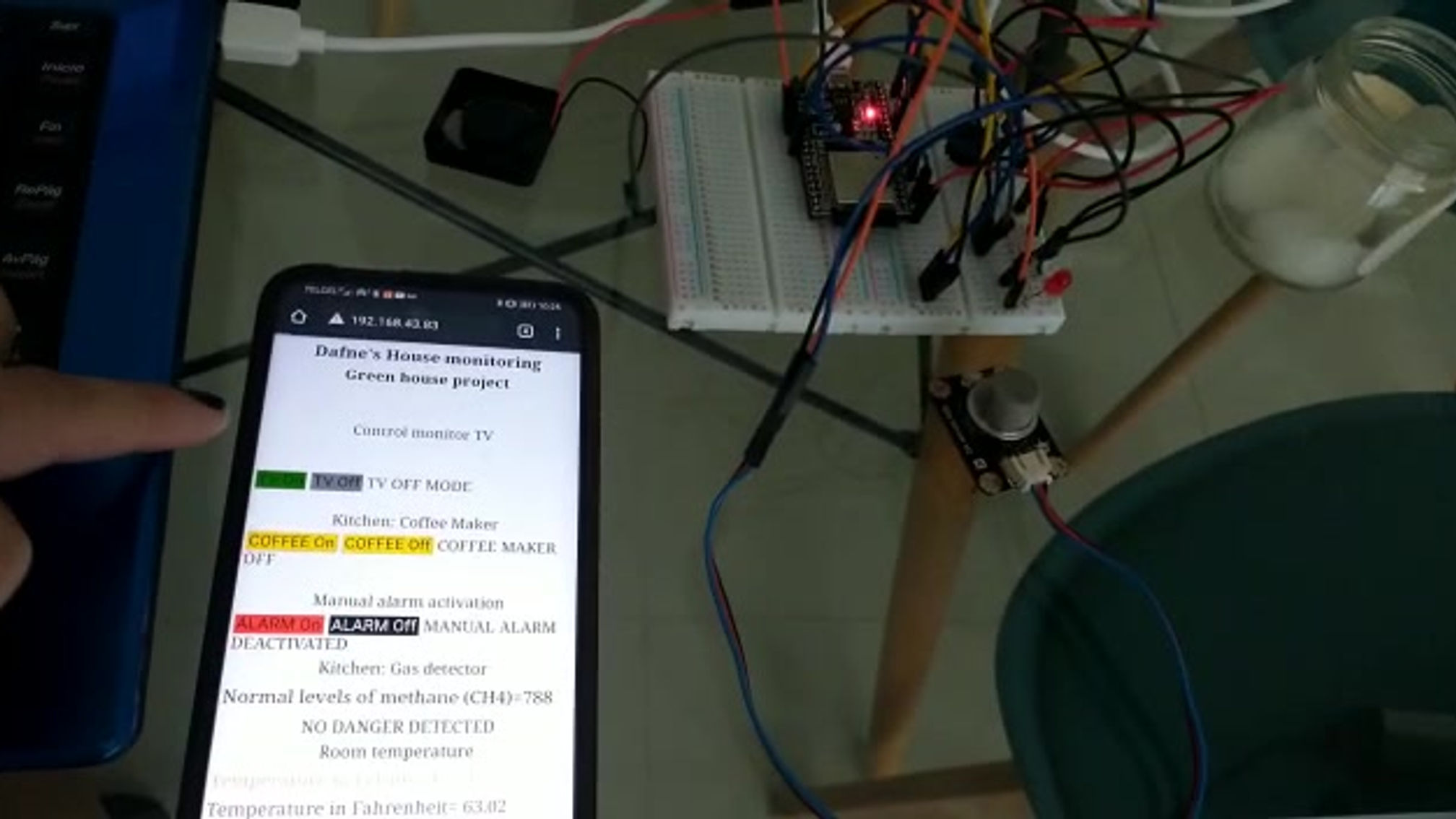